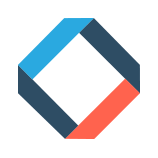
A colleague is working in a small software development company (twenty employees). He's a part of a team of five developers who work on the leading product of the company.
For a few years, the founder was unhappy about the technical skills of the employees, and he recently hired a senior develop...