0
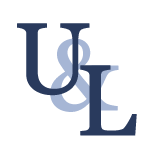
One of our accounts on a centos 7 machine experienced very slow disk read/write.
id uid=1007(test.dd) gid=1001(xxx) groups=1001(xxx),10(wheel)
ulimit -m 100000000; sudo dd if=/dev/zero of=/tmp/test1.img bs=1G
count=1 oflag=dsync
[sudo] password for test.dd: 1+0 records in 1+0
records out 1073741...