0
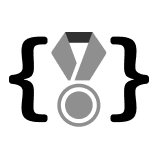
What day is it today? code-challenge
Given a date y4y3y2y1-m2m1-d2d1, output the day in the week w. 0 as Sunday, 1 as Monday, and so on. You can assume the date is valid, and every year has 12 month, every Jan,Mar,May,Jul,Aug,Oct,Dec have 31 days, every Apr,Jun,Sep,Nov have 30 days, Feb have 29 ...