6
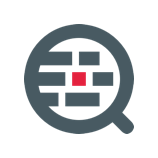
I was inspired after this thread on Stack Overflow to create a random 8 character alphanumeric password generator.
Sadly, it is closed, so I cannot provide an answer there. Anyway, here is the code. Let me know if there are any bugs/bias going on.
using System.Collections.Generic;
using System....