0
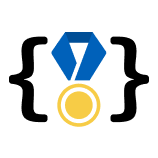
I'm not sure if this is O(n log n) as mentioned in the comments, but I used a hashing algorithm instead of my original naive nested for loop originally posted in comments. Basically, you compute the minimum of the 2 string lengths, as an optimization / exclude impossible solutions. Then you work ...