5
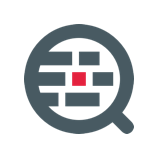
Simpler value-initialization:
UUID() : uuid(std::array<const uint8_t, 16>{
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
}) {}
Can just be:
UUID(): uuid() { }
(Well, there are many other ways. Basically, we just need to do something to it that isn't just leaving it to the default: {}...