1
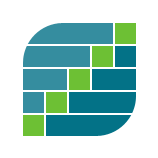
I provide here one possible answer to your question. I had to make a few assumptions, but based on your comments, I believe they are acceptable.
I will describe briefly the assumptions, the algorithm and its properties, and will leave the path for the full mathematical derivation for assertions.
...