4
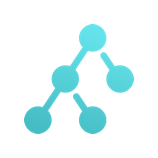
Most libraries use some variation of bottom up merge sort, but top down merge sort seems to dominate web sites and forums.
Assume reasonably optimized implementations, where a single working array is used in addition to the original array, and copy or copy back avoided in top down merge sort by ...