1
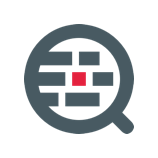
The hard part of the challenge is already done for you by Collections.shuffle(). I'll focus on style, efficiency, and elegance.
In the main() function, scanner.nextLine().replace(" ", "") is a weird thing to do. Would it be acceptable to interpret 5 0 as 50? Perhaps you meant to do String.tr...