5:52 AM
int x_move[9] = { 0, 2, 1, -1, -2, -2, -1, 1, 2 }; int y_move[9] = { 0, 1, 2, 2, 1, -1, -2, -2, -1 }; vector<vector<int>> chessmatrix(n, vector<int>(m)); for (size_t k = 0; k <= 8; k++) { int next_i = x1 + x_move[k]; int next_j = y1 + y_move[k]; if (isInside(next_i, next_j, n, m)) chessmatrix[next_i][next_j] = 1; }
2 hours later…
8:04 AM
x1--; y1--; x2--; y2--; int x_move[9] = { 0, 2, 1, -1, -2, -2, -1, 1, 2 }; int y_move[9] = { 0, 1, 2, 2, 1, -1, -2, -2, -1 }; vector<vector<int>> chessmatrix(n, vector<int>(m)); for (size_t k = 0; k <= 8; k++) { int next_i = x1 + x_move[k]; int next_j = y1 + y_move[k]; if (isInside(next_i, next_j, n, m)) chessmatrix[next_i][next_j] = 1; }
2 hours later…
10:21 AM
16
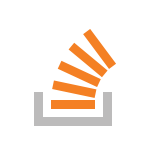
I have two arrays for a chess variant I am coding in java...I have a console version so far which represents the board as a 1D array (size is 32) but I am working on making a GUI for it and I want it to appear as a 4x8 grid, so I have a 2-dimensional array of JPanels...
Question is, is there any...
10:45 AM
And as you see, now you get a reference of current field (i, j) to it's possibly reachable fields (next_i, next_j)....
int n, m, x1, y1, x2, y2; int x_move[8] = { 2, 1, -1, -2, -2, -1, 1, 2 }; int y_move[8] = { 1, 2, 2, 1, -1, -2, -2, -1 }; cin >> n >> m >> x1 >> y1 >> x2 >> y2; vector<vector<int>> matrix(n*m, vector<int>(n*m)); for (size_t i = 0; i < n*m; i++) { for (size_t j = 0; j < n*m; j++) { for (size_t k = 0; k < 8; k++) { int next_i = i + x_move[k]; int next_j = j + y_move[k]; if (isInside(next_i, next_j, n, m)) matrix[i*m + j][next_i*m + next_j] = 1; } } }
12:03 PM
12:29 PM
int n, m, x1, y1, x2, y2; int x_move[8] = { 2, 1, -1, -2, -2, -1, 1, 2 }; int y_move[8] = { 1, 2, 2, 1, -1, -2, -2, -1 }; cin >> n >> m >> x1 >> y1 >> x2 >> y2; x1--; y1--; x2--; y2--; vector<vector<int>> matrix(n*m, vector<int>(n*m)); for (size_t i = 0; i < n*m; i++) { for (size_t j = 0; j < n*m; j++) { for (size_t k = 0; k < 8; k++) { int next_i = x1 + x_move[k]; int next_j = y1 + y_move[k]; if (isInside(next_i, next_j, n, m)) matrix[i*m + j][next_i*m + next_j] = 1;
12:58 PM
int n, m, x1, y1, x2, y2; int x_move[8] = { 2, 1, -1, -2, -2, -1, 1, 2 }; int y_move[8] = { 1, 2, 2, 1, -1, -2, -2, -1 }; cin >> n >> m >> x1 >> y1 >> x2 >> y2; x1--; y1--; x2--; y2--; vector<vector<int>> matrix(n*m, vector<int>(n*m)); for (size_t i = 0; i < n*m; i++) { for (size_t j = 0; j < n*m; j++) { for (size_t k = 0; k < 8; k++) { int next_i = x1 + x_move[k]; int next_j = y1 + y_move[k]; if (isInside(next_i, next_j, n, m)) matrix[i*m + j][next_i*m + next_j] = 1;
2:23 PM
0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
int x_move[9] = { 0, 2, 1, -1, -2, -2, -1, 1, 2 }; int y_move[9] = { 0, 1, 2, 2, 1, -1, -2, -2, -1 };
0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
3 hours later…
5:05 PM
6:01 PM
for (size_t i = 0; i < n*m; i++) { for (size_t j = 0; j < n*m; j++) { for (size_t k = 0; k < 8; k++) { int next_i = x1 + x_move[k]; int next_j = y1 + y_move[k]; if (isInside(next_i, next_j, n, m)) { cout << i*m + j << " " << next_i*m + next_j << endl; //matrix[i*m + j][next_i*m + next_j] = 1; } } } }
6:37 PM
8:24 PM
2 hours later…
10:01 PM
« first day (14 days earlier) ← previous day next day → last day (74 days later) »